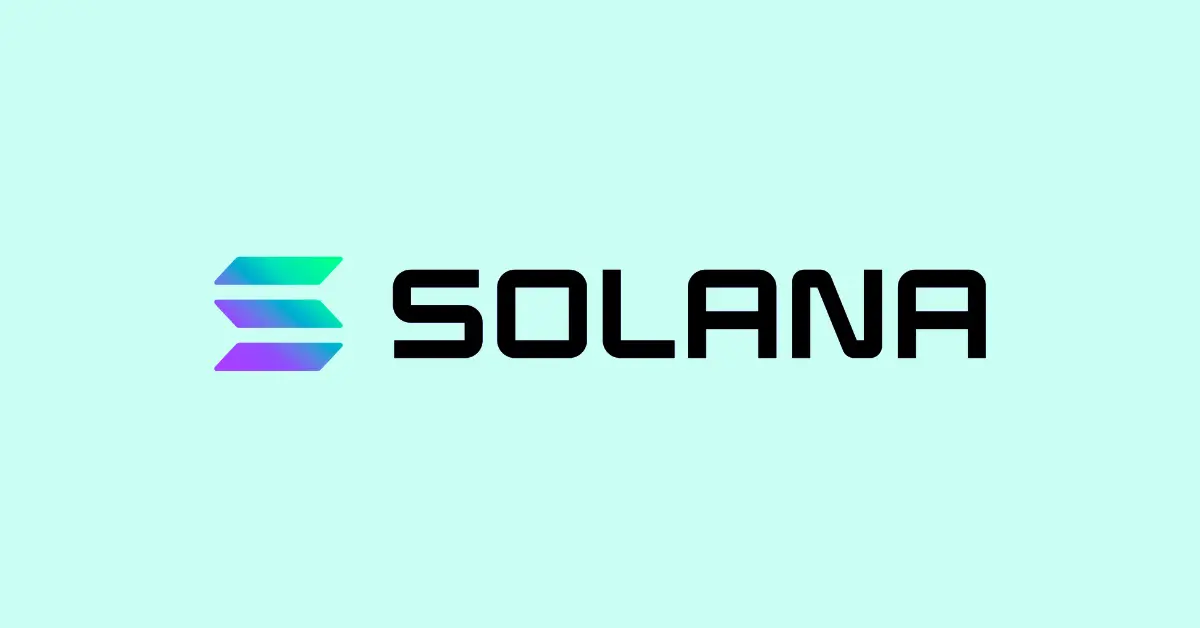
Mastering Solana Development: A Beginner's Guide
Published on 2/2/2025
Introduction to Solana
Solana has emerged as one of the most promising blockchain platforms, offering unparalleled speed and scalability. For developers looking to break into the world of blockchain, Solana presents an exciting opportunity to build cutting-edge decentralized applications (dApps) and smart contracts.
Why Choose Solana for Development?
- High throughput: Up to 65,000 transactions per second
- Low transaction costs: Fractions of a cent per transaction
- Fast finality: Transactions are confirmed in seconds
- Growing ecosystem: Rapidly expanding DeFi and NFT projects
Getting Started with Solana Development
To begin your journey in Solana development, you'll need to set up your development environment. This includes:
- Installing Rust and the Solana CLI
- Setting up a Solana wallet
- Familiarizing yourself with Solana's architecture
- Learning about Solana's programming model
While these steps can be time-consuming, our Solana Quickstart VM provides a pre-configured environment to help you start coding immediately.
Key Concepts in Solana Development
- Accounts: Solana's fundamental data structure
- Programs: Similar to smart contracts in other blockchains
- Transactions: How instructions are sent to the Solana network
- Rent: The cost of storing data on the Solana blockchain
Building Your First Solana Program
Let's walk through a simple example of a Solana program. This program will allow users to increment a counter:
use solana_program::{
account_info::AccountInfo, entrypoint, entrypoint::ProgramResult, msg, pubkey::Pubkey,
};
// Declare and export the program's entrypoint
entrypoint!(process_instruction);
// Program entrypoint's implementation
pub fn process_instruction(
program_id: &Pubkey,
accounts: &[AccountInfo],
_instruction_data: &[u8],
) -> ProgramResult {
msg!("Increment counter");
// Get the account to increment
let account = &mut accounts[0];
// Increment the counter
let mut data = account.try_borrow_mut_data()?;
let mut counter = u32::from_le_bytes([data[0], data[1], data[2], data[3]]);
counter += 1;
data[0..4].copy_from_slice(&counter.to_le_bytes());
msg!("Counter incremented to {}", counter);
Ok(())
}
This simple program demonstrates the basics of interacting with Solana accounts and modifying their data.
Next Steps in Your Solana Journey
As you continue your Solana development journey, consider exploring these advanced topics:
- Cross-program invocations
- Program Derived Addresses (PDAs)
- Token creation and management with the SPL Token program
- Building decentralized exchanges on Solana
- NFT minting and marketplaces
Conclusion
Mastering Solana development opens up a world of possibilities in the blockchain space. With its high performance and growing ecosystem, Solana is an excellent choice for developers looking to build the next generation of decentralized applications.
Ready to start your Solana development journey? Our Solana Quickstart VM provides everything you need to hit the ground running, saving you hours of setup time and potential frustration.