Mastering Solana Smart Contract Development
Published on 2/2/2025
Ready to dive into Solana smart contract development?
Get our Solana Quickstart VM and start coding in minutes!
Get Started Now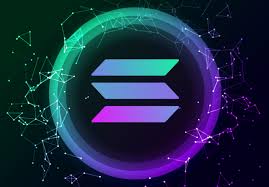
Introduction to Solana Smart Contracts
Solana smart contracts, also known as programs, are at the heart of the Solana ecosystem. They enable developers to create decentralized applications (dApps) with unprecedented speed and efficiency. In this guide, we'll explore the fundamentals of Solana smart contract development and how our Solana Quickstart VM can accelerate your learning process.
Key Concepts in Solana Smart Contract Development
- Programs: The Solana equivalent of smart contracts
- Accounts: Data storage in Solana
- Instructions: Actions that programs can perform
- Transactions: Groups of instructions executed atomically
- Borsh: The serialization format used in Solana
Getting Started with Solana Program Development
To begin developing Solana smart contracts, you'll need a properly configured environment. Our Solana Quickstart VM provides everything you need to get started quickly:
- Pre-installed Rust and Solana CLI
- Configured IDEs with Solana extensions
- Sample programs to study and modify
- Step-by-step tutorials for your first Solana program
Why Choose Our Solana Quickstart VM?
Save hours on setup and start coding immediately. Our VM comes pre-configured with all the tools you need for Solana development, plus comprehensive guides to help you along the way.
Learn MoreWriting Your First Solana Program
Let's look at a simple Solana program that increments a counter:
use solana_program::{
account_info::AccountInfo, entrypoint, entrypoint::ProgramResult, msg, pubkey::Pubkey,
};
// Declare and export the program's entrypoint
entrypoint!(process_instruction);
// Program entrypoint's implementation
pub fn process_instruction(
program_id: &Pubkey,
accounts: &[AccountInfo],
_instruction_data: &[u8],
) -> ProgramResult {
msg!("Increment counter");
// Get the account to increment
let account = &mut accounts[0];
// Increment the counter
let mut data = account.try_borrow_mut_data()?;
let mut counter = u32::from_le_bytes([data[0], data[1], data[2], data[3]]);
counter += 1;
data[0..4].copy_from_slice(&counter.to_le_bytes());
msg!("Counter incremented to {}", counter);
Ok(())
}
This program demonstrates the basic structure of a Solana program, including the entrypoint, instruction processing, and account data manipulation.
Best Practices for Solana Smart Contract Development
- Optimize for low compute and memory usage
- Use program derived addresses (PDAs) for flexible data storage
- Implement proper error handling and input validation
- Leverage Solana's parallel execution model for scalability
- Follow Solana's security best practices to protect user funds
Advanced Topics in Solana Smart Contract Development
As you progress in your Solana development journey, you'll encounter more advanced concepts:
- Cross-program invocations (CPIs)
- Token creation and management with SPL Token
- Implementing complex financial protocols
- Integrating with Solana's Serum DEX
- Optimizing programs for maximum throughput
Accelerate Your Learning
With our Solana Quickstart VM, you'll have access to advanced tutorials and sample projects covering these topics and more. Start building complex Solana applications faster than ever before.
Get Your VM NowConclusion: Your Journey to Solana Mastery
Solana smart contract development offers exciting opportunities for builders in the blockchain space. With its high performance and growing ecosystem, Solana is an excellent platform for creating the next generation of decentralized applications.
By using our Solana Quickstart VM, you can bypass the complex setup process and focus on what really matters: learning and building. Whether you're a beginner taking your first steps into blockchain development or an experienced developer looking to expand your skills, our VM provides the perfect starting point for your Solana journey.